What’s the difference between SharedFlow and StateFlow? That’s actually a very common interview question. And here’s how you can answer it:
Both SharedFlow
and StateFlow
are hot streams, but they serve slightly different purposes. Let’s answer with practical examples to better understand their use cases.
SharedFlow
SharedFlow
is like a broadcast channel. It can emit multiple values, and multiple collectors can listen to those values. It doesn’t have an initial value, but you can set how many values you want to replay to new collectors.
Example: Imagine a chat application where users can send messages. When a user sends a message, all online users should receive it. SharedFlow
can be used to broadcast these messages to all online users.
val messagesFlow: SharedFlow<String> = MutableSharedFlow()
// User A sends a message
launch {
messagesFlow.emit("Hello from User A!")
}
// User B and User C are listening to the messages
launch {
messagesFlow.collect { message ->
println("User B received: $message")
}
}
launch {
messagesFlow.collect { message ->
println("User C received: $message")
}
}
StateFlow
StateFlow
is a state holder. It always has a value (initial or updated), and it emits the latest value to its collectors. It’s useful when you want to represent a piece of state that can change over time.
Example: Consider a music player app. The player has a state (playing, paused, stopped). You can use StateFlow
to represent and observe the current state of the player.
val playerState: StateFlow<PlayerState> = MutableStateFlow(PlayerState.STOPPED)
// Somewhere in the app, the state changes
launch {
playerState.value = PlayerState.PLAYING
}
// Another part of the app observes the player's state
launch {
playerState.collect { state ->
when (state) {
PlayerState.PLAYING -> println("Music is playing!")
PlayerState.PAUSED -> println("Music is paused!")
PlayerState.STOPPED -> println("Music is stopped!")
}
}
}
In this example, whenever the player’s state changes, the observing part of the app will react accordingly.
While both SharedFlow
and StateFlow
can be used to emit and collect values, SharedFlow
is more general and can be used for broadcasting multiple values to multiple collectors. In contrast, StateFlow
is specialized for representing and observing state changes.
In the context of Android development, StateFlow
is particularly beneficial when used with Jetpack Compose and ViewModels. It provides a seamless way to observe and react to state changes in the UI, ensuring that the view layer always reflects the latest application state.
tl;dr: SharedFlow
broadcasts multiple values to many collectors, like chat messages to users. StateFlow
holds and emits the latest state, like a music player’s status. Both are hot streams with distinct use cases.
Comprehensive guide to Android Interview
Android Interview Pro
Ready to ace your Android developer interview? Get your hands on our comprehensive eBook today and start preparing with confidence.
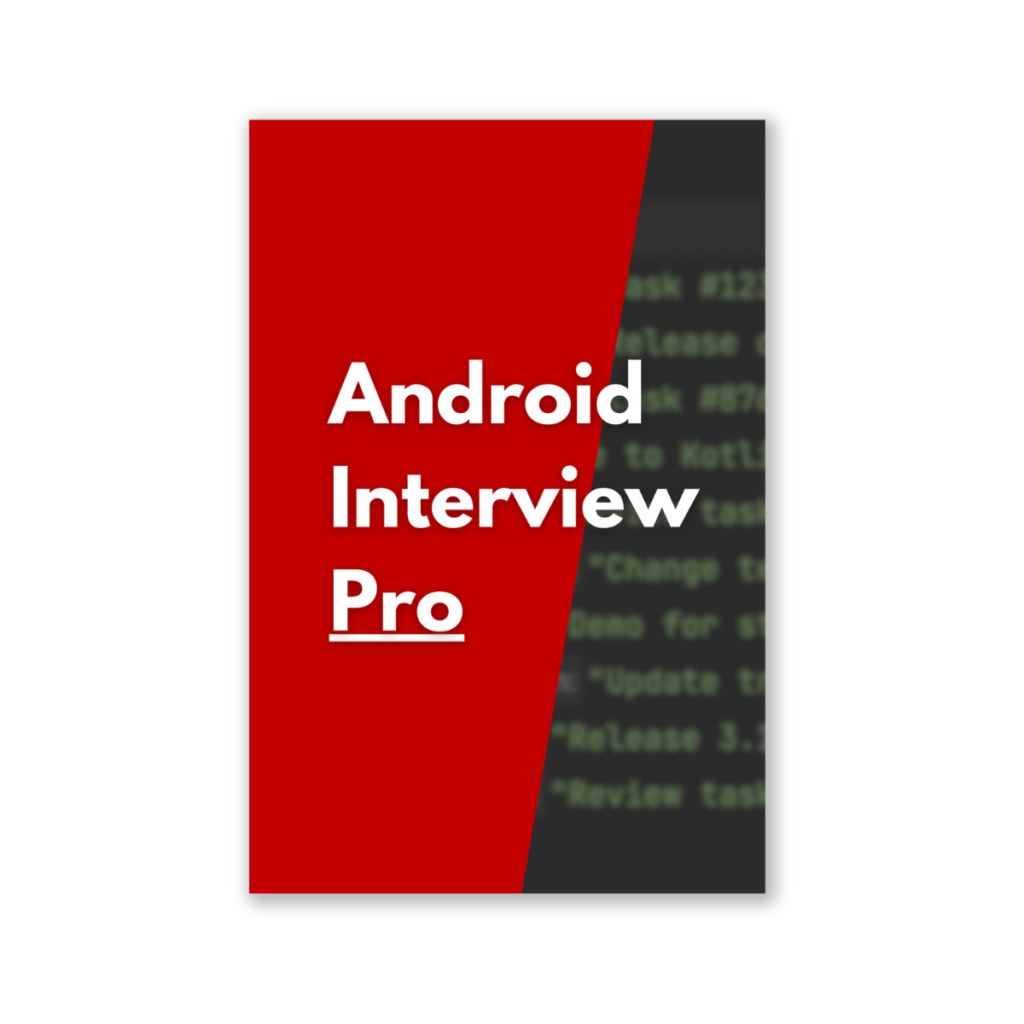